C# - Gestione dei file
Questo script permette la gestione dei file in C# in modo rapido e semplice:
<%@ Page Language="C#" Debug="true"%> <%@ import Namespace="System.IO" %> <script runat="server"> void Page_Load(Object sender, EventArgs e) { //Copio il file dalla root di C nella dir "prove" e sovrascrivo se già esistente if (CopyFile("c:/pippo.txt", "c:/prove/pippo.txt", true)) { myLabel.Text += "File copiato"; } //Sposto il file if (MoveFile("c:/pippo.txt", "c:/prove/pippo.txt")) { myLabel.Text += "File spostato"; } //Cancello il file if (DeleteFile("c:/pippo.txt")) { myLabel.Text += "File cancellato"; } } private bool DeleteFile(string NomeFile) { if (File.Exists(NomeFile)) { File.Delete(NomeFile); return true; } else { return false; } } private bool MoveFile(string OldPathFile, string NewPathFile) { if (File.Exists(OldPathFile) && File.Exists(NewPathFile)==false) { File.Move(OldPathFile, NewPathFile); return true; } else { return false; } } bool CopyFile(string OldPathFile, string NewPathFile, bool FileOverWrite) { if (File.Exists(OldPathFile)) { File.Copy(OldPathFile, NewPathFile, FileOverWrite); return true; } else { return false; } } </script> <html> <head></head> <body> <asp:Label id="myLabel" runat="server" /> </body> </html>
Script ASP
-
ASP.NET
Contatori (ASP)
Chat (ASP)
Database
Date e Ore -
Email (ASP)
File ASP
Gestione banner
Grafica e Layout
Script matematici
Articoli ASP | Libri ASP | Manuale ASP
Visual Basic 6
Imparare a programmare col noto linguaggio di casa Microsoft.MS Access
Il noto database attraverso la sua interfaccia visuale ed il linguaggio SQL.MySQL
Corso completo sul famoso DBMS open-source.ASP Completo
Corso completo per imparare a fondo lŽASP con lŽutilizzo del VBScript.
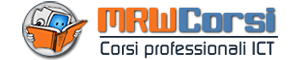